Azure Blob Lifecycle Management Part 4: Create Azure Function To Add Index Tags on Existing Blobs
- George Lin
- Oct 30, 2021
- 2 min read
Updated: Dec 3, 2021
Create a Timer Triggered .NET Azure function to add required index tags to all the existing blob objects following the same rules which are being applied to the newly uploaded/modified blobs. Enable “RunONStartup” to run the function whenever the function App restarts. Since we don’t want to run this function repeatedly, it should be disable once the task is done, but if it’s needed, the function can be invoked by a timer defined in the function code.
1. In Solution Explorer, right click on AzureBlobIndexTags, select Add, then select New Azure Function. On Add New Item – AzureBlobIndexTags window, select Azure Function, then click Add button.
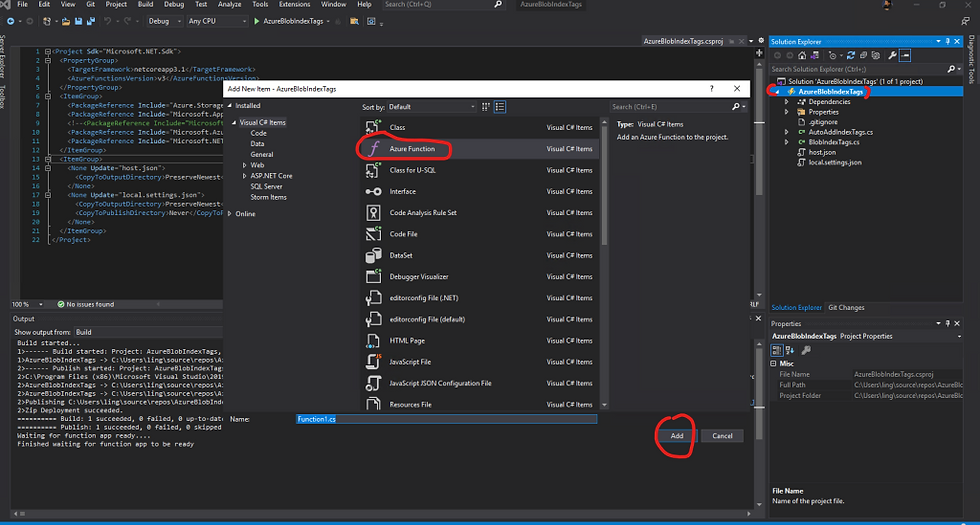
2. In Visual Studio, rename the automatically created function Function1.cs to AddIndexTagsOnExistingBlobs.cs and replace all its content with the following code block
using System;
using Microsoft.Azure.WebJobs;
using Microsoft.Extensions.Logging;
using Azure.Storage.Blobs;
using Azure.Storage.Blobs.Models;
using System.Threading.Tasks;
namespace AzureBlobIndexTags
{
public static class AddIndexTagsOnExistingBlobs
{
static string connectionString = Environment.GetEnvironmentVariable("AzureWebJobsStorage");
static string containerName = Environment.GetEnvironmentVariable("ContainerName");
[FunctionName("AddIndexTagsOnExistingBlobs")]
// [Timeout("-1")]
public async static Task RunAsync([TimerTrigger("0 5 22 31 8 6", RunOnStartup = true),] TimerInfo myTimer, ILogger log)
{
log.LogInformation($"C# Timer trigger function executed at: {DateTime.Now}");
BlobServiceClient serviceClient = new BlobServiceClient(connectionString);
BlobContainerClient container = serviceClient.GetBlobContainerClient(containerName);
var watch = new System.Diagnostics.Stopwatch();
watch.Start();
await foreach (BlobItem blobItem in container.GetBlobsAsync(BlobTraits.All))
{
BlobClient myBlob = container.GetBlobClient(blobItem.Name);
try
{
var setTagStatus = await BlobIndexTags.SetBlobIndexTagsAsync(myBlob);
if (setTagStatus == 204)
{
log.LogInformation($"Successfully sent request to set the blob index tags on {myBlob.Name} and reqeust response code is {setTagStatus}");
//Console.Write("\r{0}", new string(' ', Console.WindowWidth));
//Console.Write("\r{0}", $"Successfully sent request to set the blob index tags on {myBlob.Name} and reqeust response code is {setTagStatus}");
}
else
{
log.LogWarning($"Failed to set the blob index tags on {myBlob.Name} and reqeust response code is {setTagStatus} ");
}
}
catch (Exception ex)
{
log.LogWarning($"{ex.Message}");
log.LogWarning($"{ex.StackTrace}");
}
}
watch.Stop();
Console.WriteLine($"Execution Time: {watch.ElapsedMilliseconds} ms");
log.LogInformation($"Execution Time: {watch.ElapsedMilliseconds} ms");
}
}
}
3. Add the following two lines in local.settings.json file to disable the function AutoAddIndexTags so that we can test to run this new function AddIndexTagsOnExistingBlobs alone in the local runtime environment.
“AzureWebJobs.AddIndexTagsOnExistingBlobs.Disabled”: false, “AzureWebJobs.AutoAddIndexTags.Disabled”: true,
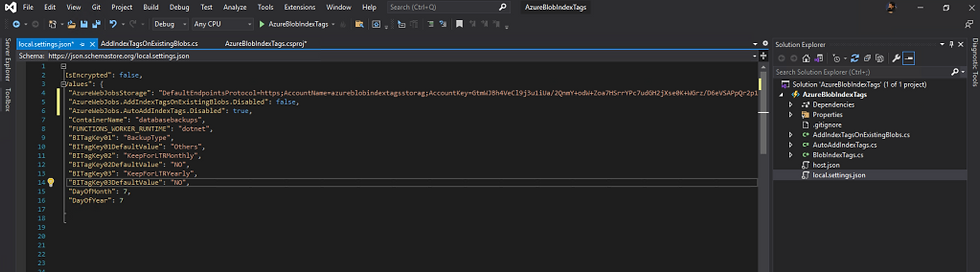
4. Manually run AzureBlobIndexTags in Visual Studio and observe the func.exe (Azure Functions Core Tools) window to verify the function run successfully and send request to set the index tags on all the existing blobs in the container.

5. Stop the function app AzureBlobIndexTags.
6. In Solution Explorer, right click on AzureBlobIndexTags, select Publish, then click Publish.
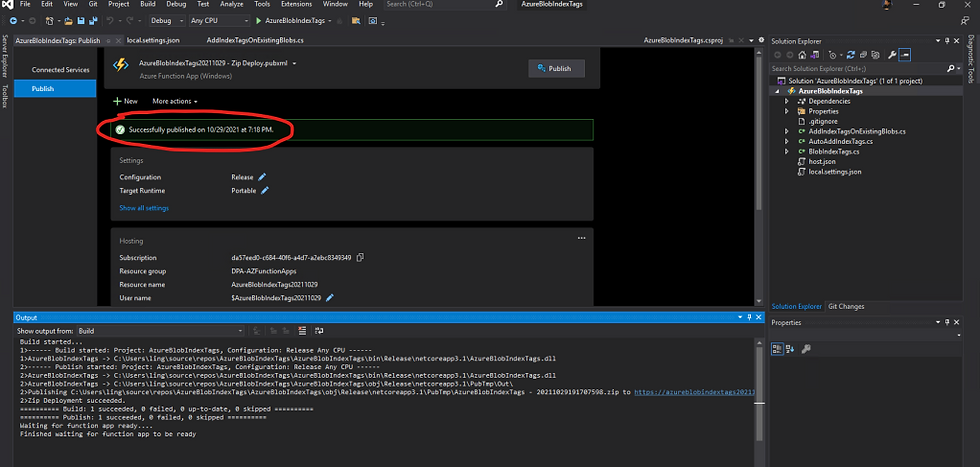
7. Login Azure portal, find the function AddIndexTagsOnExistingBlobs, go the function Monitor window, click on the latest invocation trace to show the Invocation Details window. As you can see, the function has been triggered and ran successfully.

We now have successfully created and deployed the C# Azure function AddIndexTagsOnExistingBlobs in the function App AzureBlobIndexTags20211029 in Azure.
Comments